Create iOS interactive push
iOS 8 introduced interactive notifications, allowing users to take actions directly from the notification banner. Pushwoosh now offers iOS Categories, enabling you to create custom buttons within the Pushwoosh Control Panel. Learn more
When your application calls registerDevice
, Pushwoosh API returns the response that contains a list of available Categories with their IDs and details for each button as follows:
{ "status_code": 200, "status_message": "OK", "response": { "iosCategories": [ { "categoryId": 65, "buttons": [ { "id": 0, "label": "Rate", "type": "1", "startApplication": 1 }, { "id": 1, "label": "Later", "type": "0", "startApplication": 0 } ] } ] }}
These Categories are now available on the device, so they can be properly displayed when a message arrives and your application is not running in the foreground.
In order to send your push with a category from the Pushwoosh Journey, simply select it in the iOS platform settings while composing your message. In case you are sending your pushes remotely through Pushwoosh API, in the createMessage
requests you should use the ios_category
parameter with a corresponding Category ID as a value:
{ "categoryId": 65 // Optional. String value. iOS8 category ID from Pushwoosh}
When a push message containing a category ID arrives, Pushwoosh SDK displays the notification with a set of buttons this category contains.
Buttons & actions in Pushwoosh iOS SDK
Anchor link toIn order to perform various actions upon opening an app, you should create a custom UNUserNotificationCenterDelegate
implementation and override its didReceiveNotificationResponse
method:
CustomDelegate
Anchor link toclass CustomDelegate: NSObject, UNUserNotificationCenterDelegate { func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) { let identifier = response.actionIdentifier let category = response.notification.request.content.categoryIdentifier
if category == "10212" { if identifier == "1" { // DO SOMETHING } else { // DO SOMETHING ELSE } }
completionHandler() }}
Where identifier
is a button ID, and category
is derived from the notification payload.
Then, create an instance of this class and pass it to Pushwoosh SDK using the proxy method:
AppDelegate
Anchor link tofunc application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool { Pushwoosh.sharedInstance().registerForPushNotifications()
let customDelegate = CustomDelegate() Pushwoosh.sharedInstance().notificationCenterDelegateProxy?.add(customDelegate)
return true}
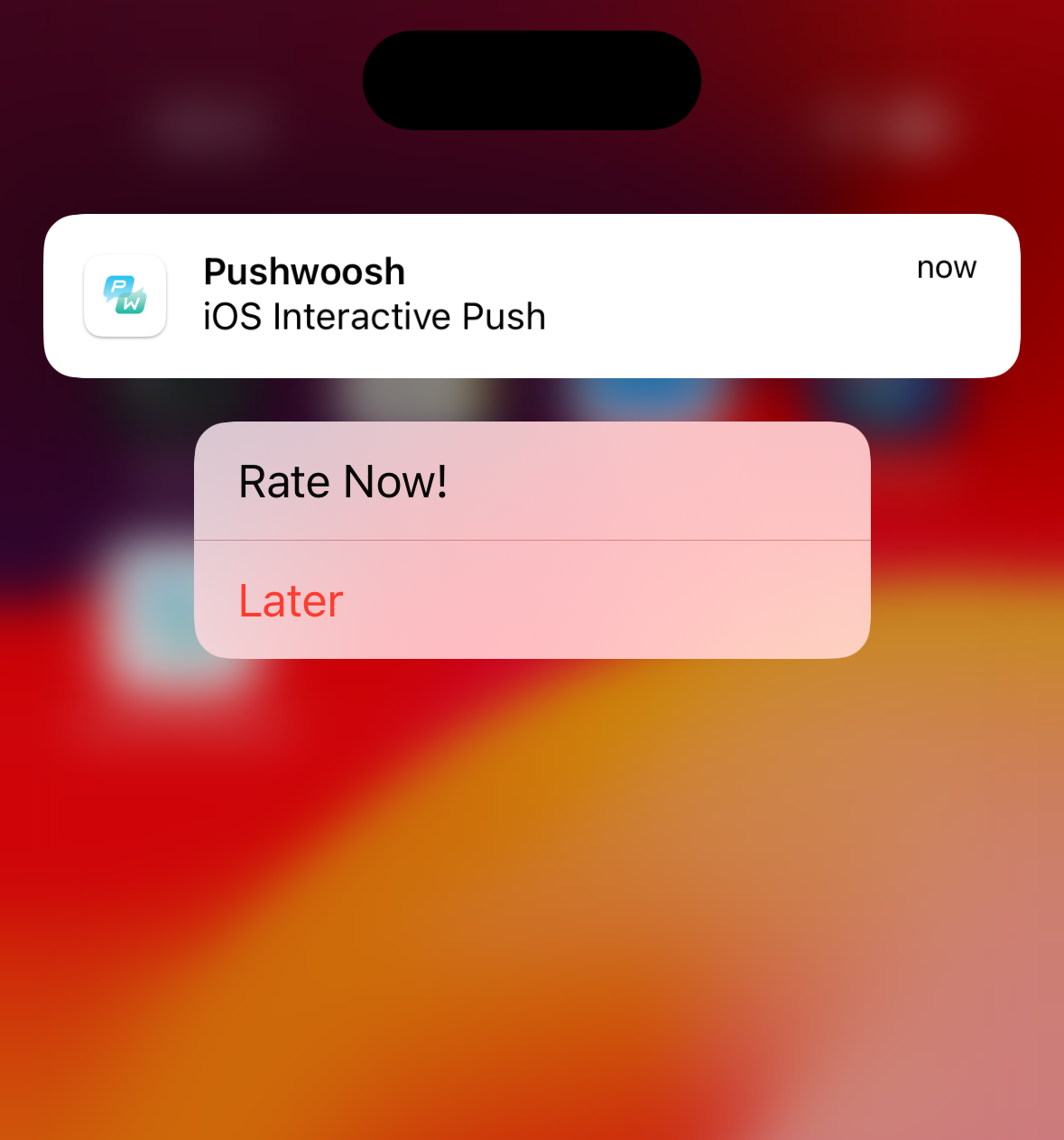