Chrome and Firefox web push for HTTP websites
Configuration
Section titled “Configuration”- Select the Web push option while creating a project.
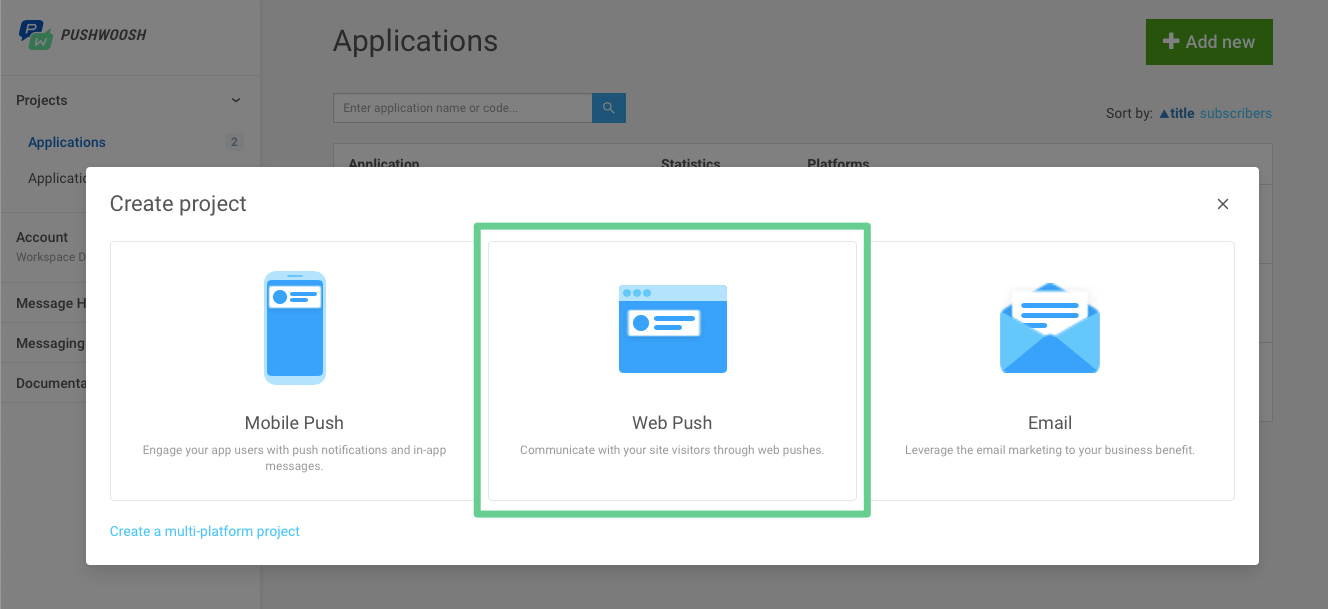
2. Choose http, enter your site URL and project name.
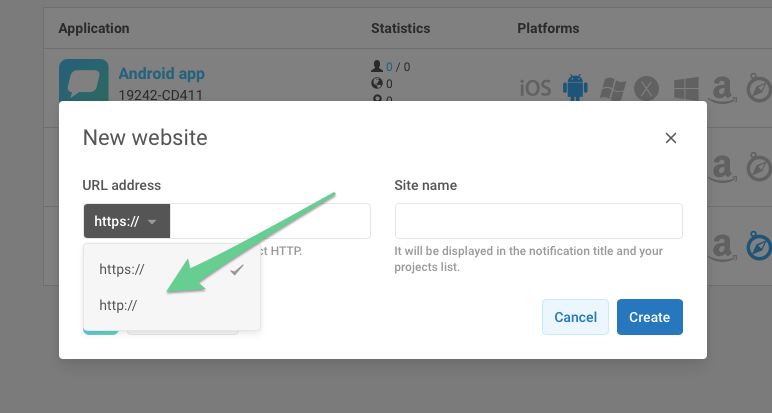
3. Configure both Chrome and Firefox with your FCM project credentials.
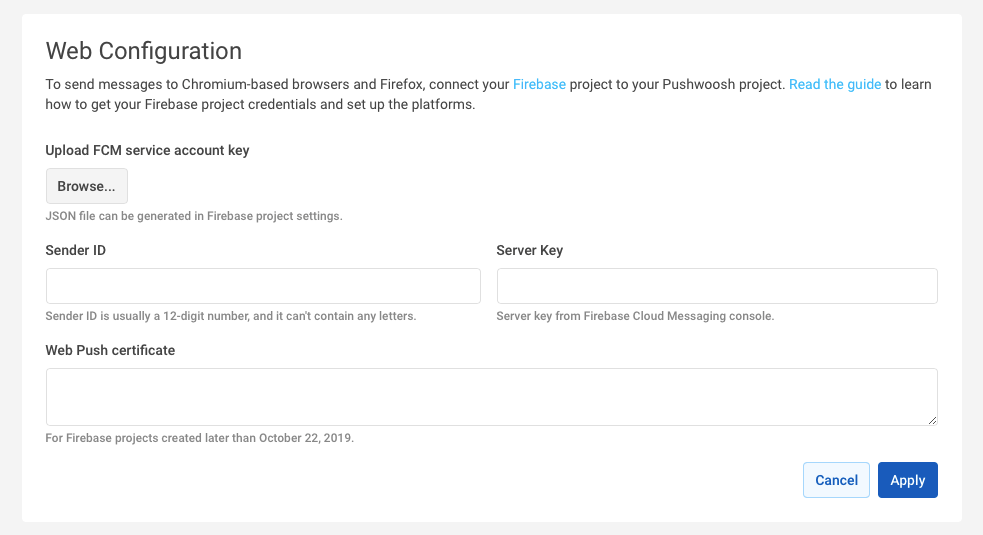
Integration
Section titled “Integration”1. Create pushwoosh-web-pushes-http-sdk.js file in the root directory of your website with the following content:
var pushwoosh = { PUSHWOOSH_APPLICATION_CODE: 'XXXX-XXXX', PUSHWOOSH_APPLICATION_CODE_GET_PARAMETER: 'pw_application_code', init: function (applicationCode) { this.PUSHWOOSH_APPLICATION_CODE = applicationCode; window.addEventListener('message', this.pwReceiveMessage, false); }, tryInitUsingGetParameter: function () { var applicationCode = this.getQueryVariable(this.PUSHWOOSH_APPLICATION_CODE_GET_PARAMETER); console.log(applicationCode); if (applicationCode) { this.init(applicationCode); } }, pwReceiveMessage: function (event) { if (event.data == 'allowPushNotifications') { localStorage.setItem('pwAllowPushNotifications', true); } }, isBrowserChrome: function () { return navigator.userAgent.toLowerCase().indexOf('chrome') > -1; }, isBrowserFirefox: function () { return navigator.userAgent.toLowerCase().indexOf('firefox') > -1; }, isBrowserSafari: function () { return navigator.userAgent.toLowerCase().indexOf('safari') > -1 && !this.isBrowserChrome(); }, isBrowserSupported: function () { return this.isBrowserChrome() || this.isBrowserFirefox(); }, subscribeAtStart: function () { if (this.isBrowserSupported()) { if (null === localStorage.getItem('pwAllowPushNotifications')) { this.showSubscriptionWindow(); } } }, isSubscribedForPushNotifications: function () { return true == localStorage.getItem('pwAllowPushNotifications'); }, showSubscriptionWindow: function () { if (this.isBrowserSupported()) { var windowWidth = screen.width / 2; var windowHeight = screen.height / 2;
var windowLeft = screen.width / 2 - windowWidth / 2; var windowRight = screen.height / 2 - windowHeight / 2;
var URL = 'https://' + this.PUSHWOOSH_APPLICATION_CODE + '.chrome.pushwoosh.com/'; var pwSubscribeWindow = window.open(URL, '_blank', 'width=' + windowWidth + ',height=' + windowHeight + ',resizable=yes,scrollbars=yes,status=yes,left=' + windowLeft + ',top=' + windowRight); } }, getQueryVariable: function (variable) { // document.currentScript won't work if this code is called from function in event lister if (document.currentScript) { var urlParts = document.currentScript.src.split('?'); if (typeof urlParts[1] !== 'undefined') { var vars = urlParts[1].split('&'); for (var i = 0; i < vars.length; i++) { var pair = vars[i].split('='); if (pair[0] == variable) { return pair[1]; } } } } else { console.error('Cannot get current script address'); } return null; }};pushwoosh.tryInitUsingGetParameter();
2. Include the previous file to your website and initialize it using the application code instead of XXXXX-XXXXX
<!--[if !IE]><!--> <script src="/pushwoosh-web-pushes-http-sdk.js?pw_application_code=XXXXX-XXXXX"></script><!--<![endif]-->
3. In order to create a Push Subscription button use the following:
<button onclick="pushwoosh.showSubscriptionWindow()">Subscribe to push notifications</button>
4. Alternatively, if you want Notification Subscriptions to pop up automatically (opposed to #4 above), use the following:
<script>pushwoosh.subscribeAtStart();</script>
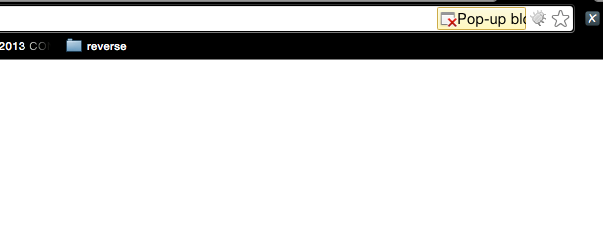
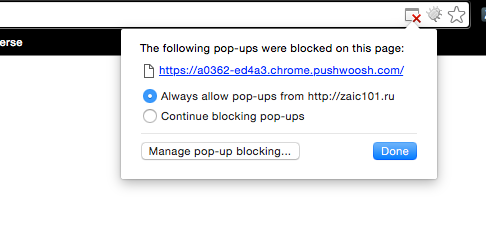
As a result, the user will be prompted to subscribe for push notifications from the website:
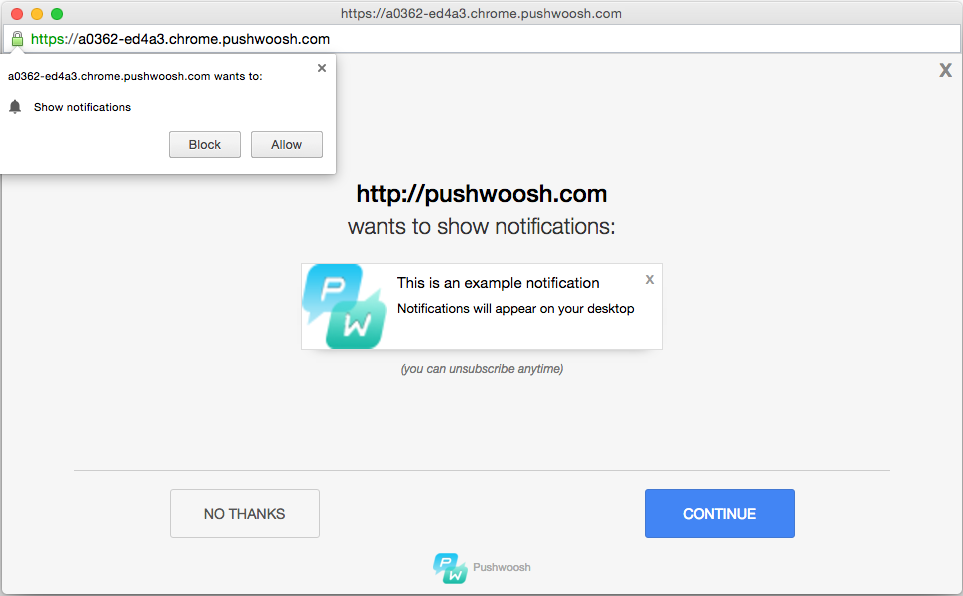