Setting up badges for Flutter
1. Open your iOS project located in your_project/ios/Runner.xcworkspace and create NotificationServiceExtension:
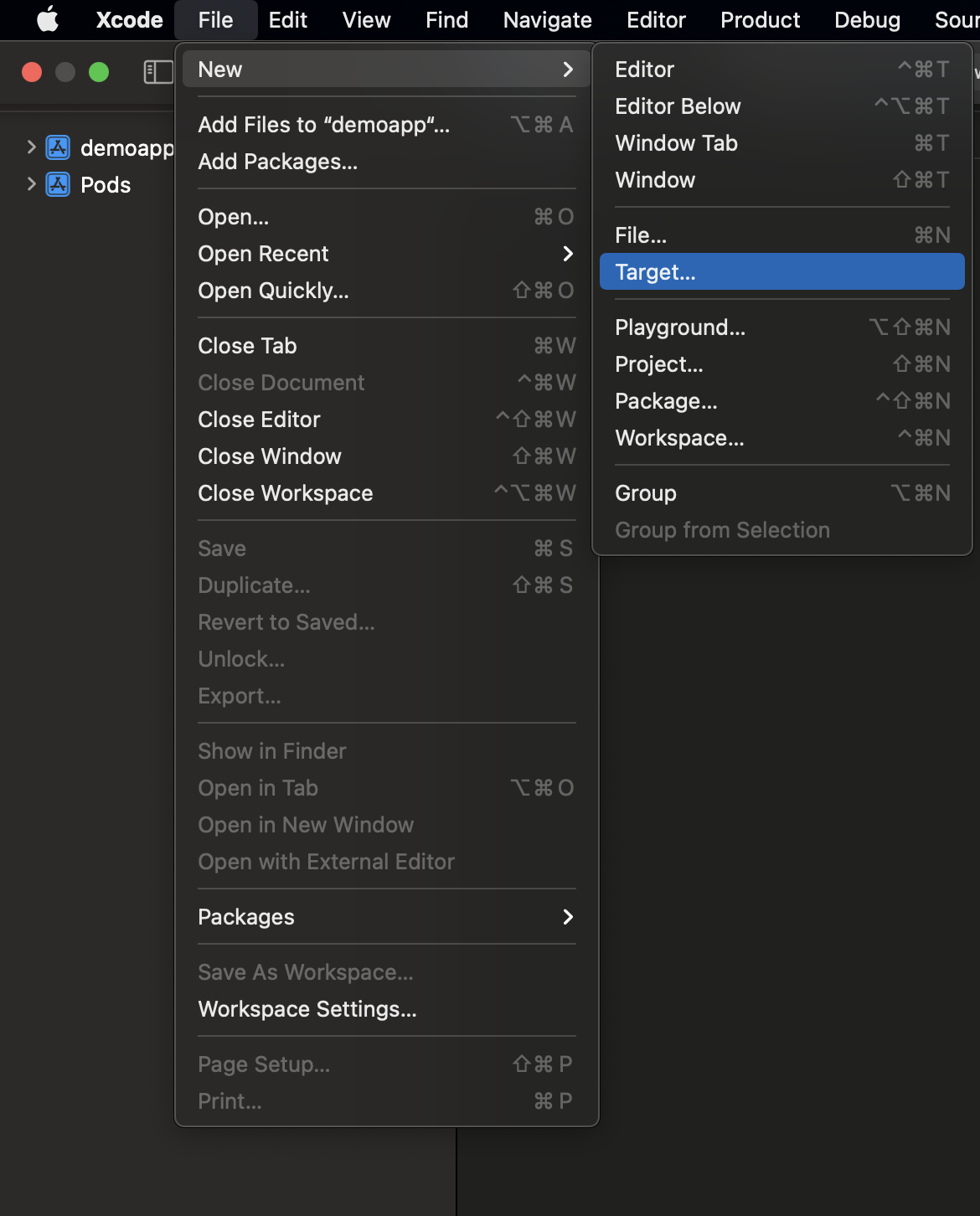
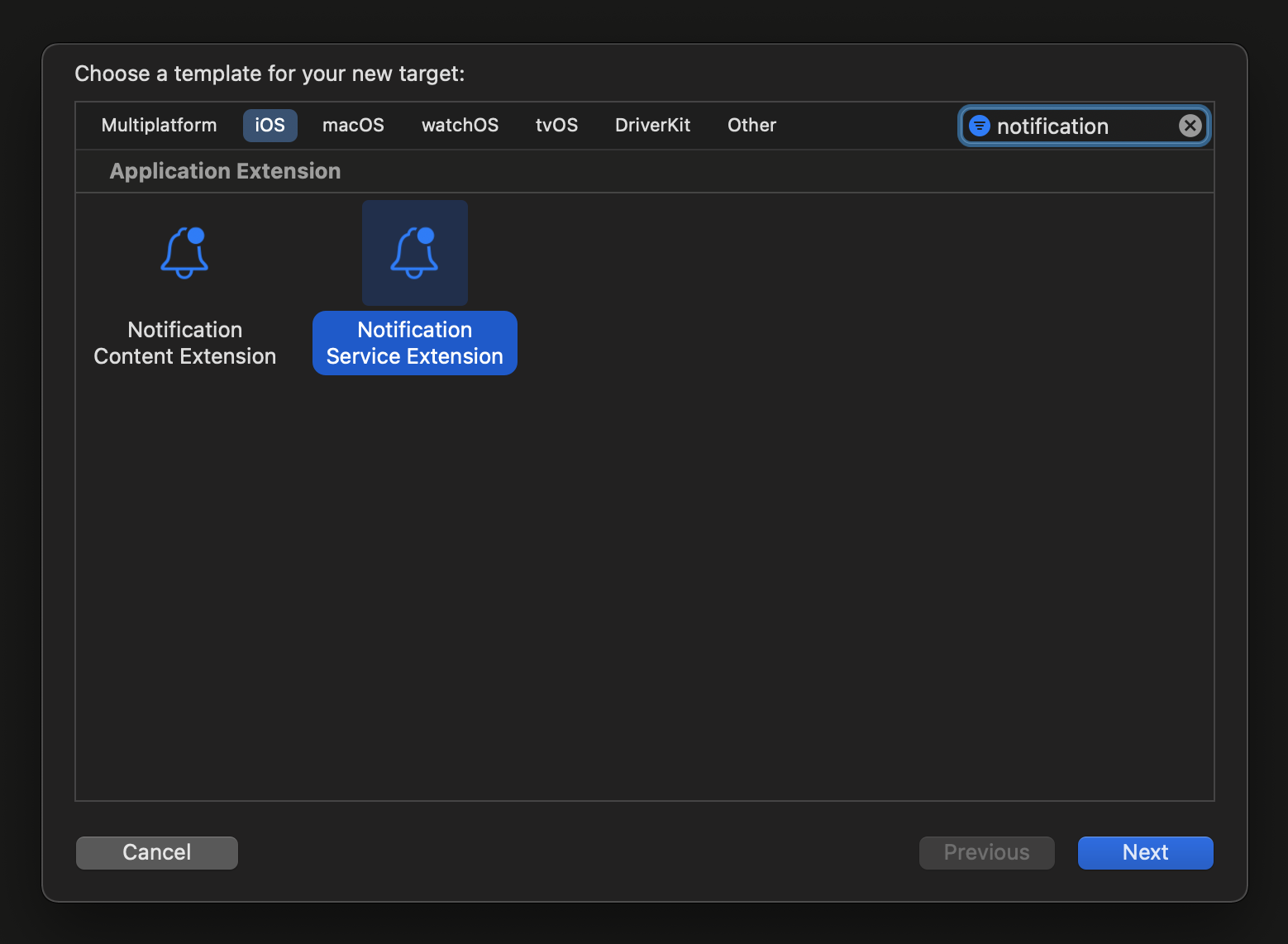
2. Open Podfile (located in your_project/ios/Podfile) and add PushwooshXCFramework dependency fo NotificationServiceExtensionTarget:
target ‘NotificationService’ do pod ‘PushwooshXCFramework’, ‘>=6.5.0’end
3. Install pods via Terminal:
cd ios && pod install
4. Close and reopen your Xcode project.
5. Make sure that your Deployment target matches the one in the Runner target; otherwise, you might face an issue when building your app (e.g., if you specify iOS 10.0 in Runner and iOS 15.5 in NotificationService targets).
6. Add App Groups capability to both Runner and NotificationService targets and add a new group with the same name for both targets:
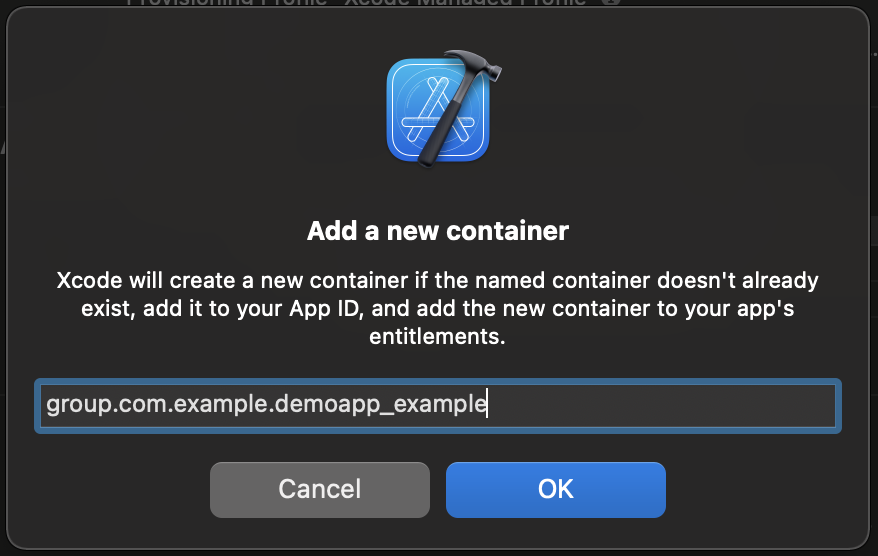

7. Add PW_APP_GROUPS_NAME info.plist flag to both Runner and NotificationService targets with the group name as its string value:

8. Go to NotificationService.m and replace its code with the following:
#import “NotificationService.h”#import <Pushwoosh/PWNotificationExtensionManager.h>
@interface NotificationService ()<strong>@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);</strong>@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;@end
@implementation NotificationService- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler { self.contentHandler = contentHandler; self.bestAttemptContent = [request.content mutableCopy]; [[PWNotificationExtensionManager sharedManager] handleNotificationRequest:request contentHandler:contentHandler];}- (void)serviceExtensionTimeWillExpire { // Called just before the extension will be terminated by the system. // Use this as an opportunity to deliver your “best attempt” at modified content, otherwise the original push payload will be used. self.contentHandler(self.bestAttemptContent);}@end
That’s it!
Share your feedback with us
Your feedback helps us create a better experience, so we would love to hear from you if you have any issues during the SDK integration process. If you face any difficulties, please do not hesitate to share your thoughts with us via this form.