Custom subscription Popup
Custom Subscription Popup is a pop-up window displayed on the web page and suggesting users to subscribe to web push notifications. It’s implemented with Pushwoosh WebSDK and allows to overcome the browser restrictions on a native subscription prompts displaying. A native subscription popup can be shown to a particular user only twice. If a user doesn’t allow push notifications and closes the native subscription popup two times in a row, it won’t be shown again and push notifications are considered blocked. A Custom Subscription Popup in its turn can be displayed as many times as needed unless a user subscribes to or blocks push notifications explicitly.
A Custom Subscription Popup can contain any text explaining the advantages of subscription to push notifications and two customizable buttons — a one for an “Ask later” option and a one for “Subscribe.”
Once a user presses a “Subscribe” button, a native subscription popup appears.
When a user presses an “Ask Later” button or simply closes the window, the Custom Subscription Popup will appear again in a specified time (by default, in a week after; a delay to retry can be customized via the “retryOffset” parameter).
Implementation
To enable and customize a Custom Subscription Popup, use the following script while initializing Pushwoosh WebSDK:
Pushwoosh.push('init', { . . ., subscribePopup: { enable: true, // (boolean) popup activation text: 'Text on popup', // (string) a text to display on the popup askLaterButtonText: 'Not now', // (string) custom text for the “Ask later” button confirmSubscriptionButtonText: 'Subscribe', // (string) custom text for the “Subscribe” button iconUrl: 'https://...', // (string) custom icon URL delay: 60, // (integer) a delay between the page loading and popup appearance retryOffset: 604800, // (integer) an offset in seconds to display the popup again overlay: false, // (boolean) enables page overlaying when popup is displayed position: 'top', // (string) position on the page. Possible values: ’top' | 'center' | 'bottom’
bgColor: '#fff', // (string) popup’s background color borderColor: 'transparent', // (string) popup’s border color boxShadow: '0 3px 6px rgba(0,0,0,0.16)', // (string) popup’s shadow
textColor: '#000', // (string) popup’s text color textSize: 'inherit', // (string) popup’s text size fontFamily: 'inherit', // (string) popup’s text font
subscribeBtnBgColor: '#4285f4', // (string) “Subscribe” button’s color subscribeBtnTextColor: '#fff', // (string) “Subscribe” button text’s color
askLaterBtnBgColor: 'transparent', // (string) “Ask later” button’s color askLaterBtnTextColor: '#000', // (string) “Ask later” button text’s color
theme: 'material' // or 'topbar'. A popup theme, see the details below }});
Themes
By default, the Subscription Popup uses a ‘material’ theme and overlays some part of a screen when shown, thus hiding some content from a website visitor.
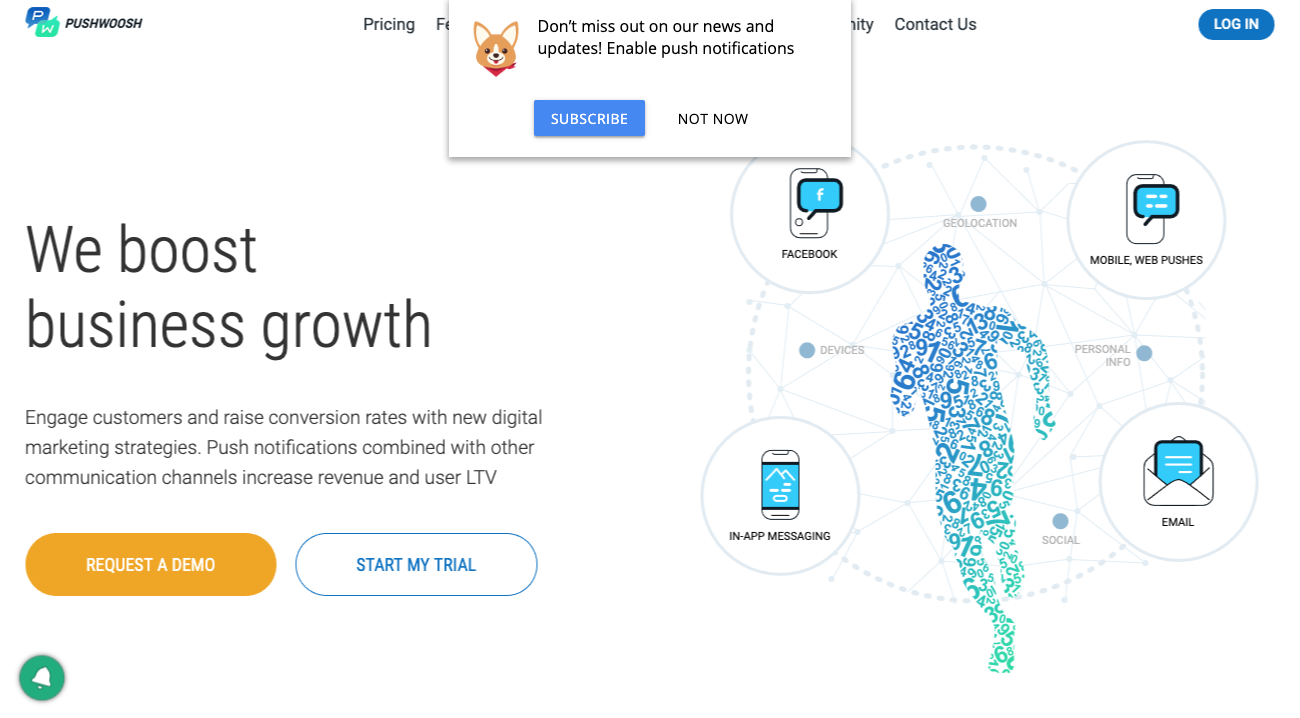
To avoid hiding valuable content by the Subscription Popup, use the ‘topbar’ theme so that the popup is shown as a narrow bar on the top of the screen.
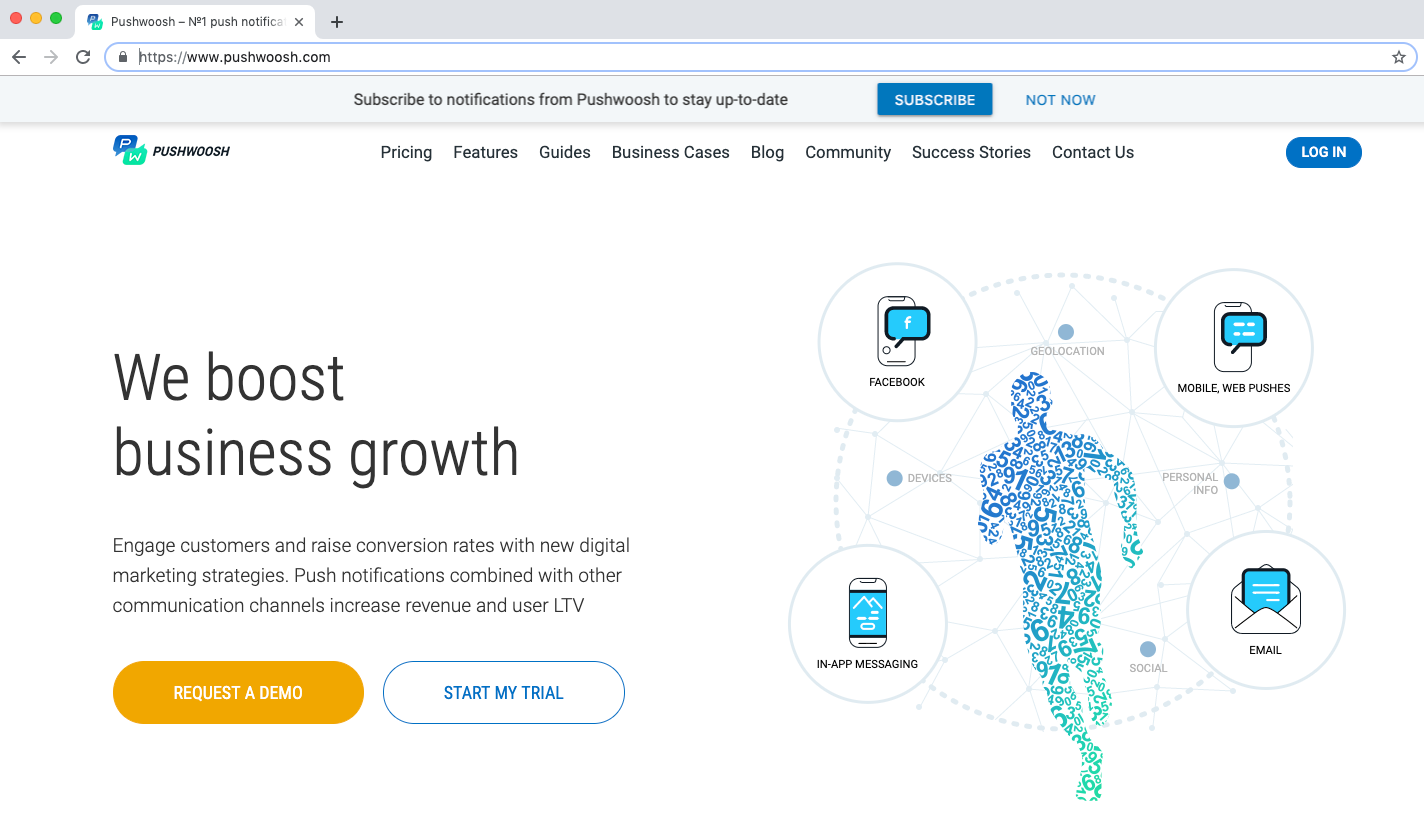